Microsoft Dynamics AX Testing
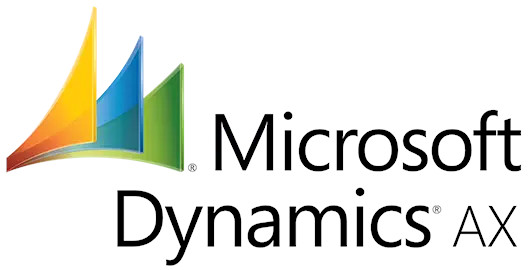
Microsoft Dynamics AX (Dynamics 365 Finance and Operations) is a robust ERP software for medium and large organizations that provides centralized finance management with real-time insights and embedded data analytics for intelligent decision-making. This versatile solution covers various aspects, including cost administration, production control, capital management, ledger, and inventory management.[raw]
Unit Testing
Unit testing is a software testing method that focuses on verifying the correctness of individual units or components of a software application in isolation. A unit refers to the smallest testable part of the software, such as functions, methods, classes, or modules. Developers write and execute unit tests during development to ensure that each code unit behaves as expected and produces the correct output for a given set of inputs.
- Unit Test Framework
- xUnit
Let’s review each framework and understand how to perform unit testing.
Unit Test Framework
The Unit Test framework is a built-in framework tightly integrated into Dynamics AX’s MorphX IDE. This integration allows for Test Driven Development (TDD), meaning unit test cases can be developed together with specific feature development. Usually, a unit test class extends the SysTestCase
class. The SysTestCase
class defines the structure for tests in the Unit Test framework.
The Unit Test framework uses X++, the default language for developing and testing Dynamics AX applications. Typically, the unit test case has a name similar to the class it tests, followed by the word Test to associate it with the test case. The Unit Test framework also offers reporting options known as listeners, where a default listener is a database during a test case run. In the Unit Test parameters form, you can add other listeners- Infolog, Infolog (result only), Print window, Progress bar, Text file, and XML file.
Class EmployeeTest extends SysTestCase { Employee employee; public void setUp() { // Create an instance of the Employee class for each test method. employee = new Employee("your name"); super(); } void testName() { // Verify that the name is set. this.assertEquals("your name", employee.name()); } }
xUnit
xUnit is a free and open-source unit testing tool for the .NET Framework. It belongs to the xUnit testing family and is inspired by the JUnit framework for Java. Developers prefer xUnit because of its simplicity, clarity, and extensibility when creating unit tests. Typically, unit test cases are written in C# using xUnit.
using Xunit; public class StringUtilsTests { [Fact] public void TestReverseString() { // Arrange StringUtils stringUtils = new StringUtils(); string input = "hello"; // Act string result = stringUtils.ReverseString(input); // Assert string expected = "olleh"; Assert.Equal(expected, result); } [Fact] public void TestToUpperCase() { // Arrange StringUtils stringUtils = new StringUtils(); string input = "hello"; // Act string result = stringUtils.ToUpperCase(input); // Assert string expected = "HELLO"; Assert.Equal(expected, result); } }
Integration Testing
Integration testing focuses on verifying the interaction and proper functioning of different components, modules, or systems when they are integrated. The main objective of integration testing is to ensure that the integrated components work together as expected and that any interface or communication points between them function correctly.
In Dynamics AX, you can perform different integration testing, such as component and data integration. The following tools are used for integration testing of the Dynamics AX application.
- Data Task Automation Framework
- Acceptance Test Library
Data Task Automation Framework
The Data Management Framework, or Data Task Automation Framework, makes importing, exporting, and synchronizing data between the application and external sources easy. It is essential for data integration testing, as it allows you to check how data is exchanged and processed between different systems. With this framework, you can repeat various data tasks and ensure the accuracy of the results.
Some critical aspects of the Data Management Framework include:
- Data Import: With DMF, you can easily import data from different sources like Excel files, CSV files, or external systems. It offers a user-friendly wizard-based interface that guides you through the data import process. This interface helps map the source data to the correct fields in Dynamics AX.
- Data Export: With DMF, you can effortlessly export data from Dynamics AX to external files or systems. This feature is beneficial for generating reports, creating backups, and sharing data with external stakeholders.
- Data Synchronization: DMF allows data synchronization between Dynamics AX, which is useful when integrating with external applications or ensuring data consistency across different systems.
- Data Staging: DMF uses a staging concept to handle data before importing it into the application. This staging area lets you review and validate the data before making it official, ensuring accurate and reliable data.
- Data Packages: DMF organizes data into packages to manage and track specific data sets efficiently. You can create and execute data packages per your needs, improving organization and control of data imports and exports.
- Data Entity Framework: DMF uses the Data Entity Framework, which offers a standardized way to work with data in Dynamics 365 Finance and Operations. Data entities represent data tables or views in the application, and DMF utilizes them for managing data during import, export, and synchronization tasks.
Acceptance Test Library
The Acceptance Test Library (ATL) is a tool written in X++ and is mainly used for integration testing with several advantages:
- Helps create consistent test data, ensuring standardized testing.
- Makes test code more readable and easy to understand and maintain.
- Improves the discoverability of methods for generating test data, making it simpler to find and use the necessary data setup.
- Hides the complexity of setting up prerequisites, making test preparation easier.
- Supports high-performance test cases, ensuring efficient and practical testing.
// Create the data root node var data = AtlDataRootNode::construct(); // Get a reference to a well-known warehouse var warehouse = data.invent().warehouses().default(); // Create a new item with the "default" setup using the item creator class. Adjust the default warehouse before saving the item. var item = items.defaultBuilder().setDefaultWarehouse(warehouse).create(); // Add on-hand (information about availability of the item in the warehouse) by using the on-hand adjustment command. onHand.adjust().forItem(item).forInventDims([warehouse]).setQty(100).execute(); // Create a sales order with one line using the sales order entity var salesOrder = data.sales().salesOrders().createDefault(); var salesLine = salesOrder.addLine().setItem(item).setQuantity(10).save(); // Reserve 3 units of the item using the reserve() command that is exposed directly on the sales line entity salesLine.reserve().setQty(3).execute(); // Verify inventory transactions that are associated with the sales line using the inventoryTransactions query and specifications salesLine.inventoryTransactions().assertExpectedLines( invent.trans().spec().withStatusIssue(StatusIssue::OnOrder).withInventDims([warehouse]).withQty(-7), invent.trans().spec().withStatusIssue(StatusIssue::ReservPhysical).withInventDims([warehouse]).withQty(-3));
End-to-end Testing
For Dynamics AX applications, end-to-end testing is critical as it is often used to manage complex business processes, such as finance, supply chain, and production. Also, Dynamics AX typically integrates with various external systems, such as CRM, HRM, or third-party applications. E2E testing verifies that these business processes are correctly configured and delivering the desired outcomes. Also, it validates that the data exchange and integration points are functioning correctly, maintaining data synchronization and smooth communication between systems. Let’s review commonly used tools for end-to-end testing for Dynamics AX applications.
Microsoft EasyRepro
Microsoft EasyRepro, or Easy Repro, is an open-source testing framework created by Microsoft. It efficiently automates UI testing for Microsoft Dynamics 365 and the Power Platform. It uses Selenium as its foundation and provides APIs and methods that let testers and developers interact with the application’s user interface elements through code. With EasyRepro, tasks like navigating screens, entering data, clicking buttons, and verifying results can be automated and repeated reliably. It works across different browsers and platforms, supports data-driven testing, and has a large community for support.
using Microsoft.Dynamics365.UIAutomation.Api; using Microsoft.Dynamics365.UIAutomation.Browser; using OpenQA.Selenium; class Program { static void Main(string[] args) { // Define the URL of your Dynamics 365 Finance and Operations environment var url = "https://your-d365fo-environment-url/"; // Define the login credentials for the application var username = "your-username"; var password = "your-password"; // Initialize the browser and navigate to the login page using (var xrmApp = new XrmApp(BrowserType.Chrome, url)) { xrmApp.OnlineLogin.Login(username, password); // Wait for the main page to load after login xrmApp.ThinkTime(2000); // Navigate to the desired module and page xrmApp.Navigation.OpenSubArea("Sales", "Customers"); // Wait for the page to load xrmApp.ThinkTime(2000); // Validate the presence of a specific element on the page var element = xrmApp.Entity.GetGridItem(0); if (element != null) { // The element is found, perform further actions or assertions // For example, you can click on the element or assert its content element.Click(); } else { // The element is not found, log an error or handle the absence of the element } } } }
TestModeller.io
TestModeller is a browser-based automation tool that helps model E2E scenarios as a BPMN (Business Process Model and Notation) style flowchart, simplifying complex scenarios. The tool automatically generates test cases from the model, optimizing testing for efficiency and risk coverage.
TestModeller.io offers a convenient UI Recorder as an extension for popular browsers like Google Chrome, Firefox, and Internet Explorer. The UI Recorder automatically captures tester activity on the browser and imports it into TestModeller.io, allowing for effortless test creation. The recorded tests can be executed on Google Chrome, Firefox, Microsoft Edge, and Safari, expanding compatibility and test execution capabilities.
As a drawback, TestModeller doesn’t support parallel execution, and its integration with test management or CI/CD tools is a bit tricky.
Regression Suite Automation Tool (RSAT)
RSAT, an inbuilt tool provided by Microsoft for testing Dynamics AX applications, simplifies the process by allowing users to record business tasks using the Task Recorder. These recordings can then be converted into a suite of automated tests. Each RSAT test case is based on a separate recording, and the tool generates executable files with C# code and an Excel file for test parameters.
During test execution, RSAT uses the Selenium framework to automatically start up a browser, navigate to the system, and repeat the steps from the given recording.
However, the main drawback of RSAT is its lack of reusability. Once a test is created, it cannot be used for different test suites. This limitation impacts the project timeline and may require additional resources for testing.
testRigor
The tools mentioned earlier pose challenges regarding maintenance and ease of use. Most of these tools depend on a programming language; hence, quality assurance demands resources with substantial programming skills. Moreover, the maintenance required for test scripts can significantly impact the time allocated for new script creation.
For instance, most record and playback tools cannot create reusable scripts, leading to repetitive efforts for each test case. Additionally, some tools may depend on Document Object Model (DOM) elements, making tests more susceptible to errors when DOM element properties change. These issues can contribute to a more complex and time-consuming testing process. testRigor overcomes all these hurdles with its exciting features:
- Eliminate the need for testers to know programming languages or involve programmers in testing.
- Empower manual testers, business analysts, and stakeholders to create automation scripts without technical barriers.
Use Generative AI for Test Creation: testRigor goes beyond enabling automated test script creation in plain English. It leverages powerful generative AI capabilities to automatically generate test cases with minimal user input. Users merely need to provide a brief description, and testRigor promptly generates the necessary test cases in seconds.
Supports all Major Testing Types: It is a robust testing tool encompassing many testing types – web, desktop, mobile, iOS, Android, API, visual regression, cross-browser, and cross-platform testing.
Powered by testRigor Locators: testRigor utilizes a unique approach to identifying element locators, avoiding unreliable XPaths or other selectors that may fail when DOM element properties change. This means you can indicate the position or name of the element they wish to interact with. E.g., click “Submit” button
or click "Continue" to the right of "Cancel"
. testRigor takes care of the rest, making test creation easier and resulting in fewer flaky tests.
Easy Integrations: Besides its powerful testing capabilities, testRigor provides seamless integrations with various popular CI/CD tools, test management tools, infrastructure service tools, and communication tools. This built-in integration ensures a hassle-free user experience in creating and managing testing processes.
Have a look at the top testRigor features.
open url "https://www.amazon.com" click "Sign In" enter stored value "email" into "email" click "Continue" enter stored value "password" into "password" click "Login" enter "Men's Printed Shirt" roughly to the left of "search" click "Search" click on image from stored value "Men's Printed Shirt" with less than "10" % discrepancy click "Add to Cart" click "Cart" click "Checkout"
This example shows why organizations now prefer testRigor over traditional and Selenium-based test automation tools. testRigor uses minimal and straightforward test steps, making test case creation or maintenance easy and quick.
Conclusion
Microsoft Dynamics AX is designed for industries like Service, Manufacturing, Distribution, Finance, Public, and Retail and aims to unite teams, promote growth, and enable scalability in organizations. Each testing type discussed in this article plays a vital role in mitigating risks, improving the quality of the implementation, and ensuring that Microsoft Dynamics AX operates effectively within an organization’s unique context.